How to Continue an Input Command if Input is Invalid in Java
TABLE OF CONTENT |
---|
Using Scanner to take Input from the user in Java |
Take Input of different data types from the user in Java |
Types of Input in Java |
Errors in Java |
Types of Errors in Java |
Previously, we used to take numbers, add them up and display the result.
Now we will make things a bit interesting. Instead of assigning values to the variables by ourselves, we will let the user input values for variables. And based on those input we will do our calculations.
Input refers to any information or data sent to a computer for processing.
Using Scanner to take Input from the user in Java
For this we will start with a simple program.
Problem #1
Sum and Average
Take three numbers. Add them up. Display the result. Also find the average of those 3 numbers.
Take inputs from the user.
So, if we take three variables 'i', 'j' and 'k', and assign them random values like 24, 39 and 15 correspondingly, here is the solution to the program.
Create a new class. I will name it addNav. And here goes the simple program –

public class addNav { public static void main(String[] args) { int i=24; int j=33; int k=15; int sum=i+j+k; int average=sum/3; System.out.println("The sum is : " + sum); System.out.println("The average is : " + average); } }
Now here we want the variables 'i', 'j' and 'k' to accept Input from the user.
There are many ways to take Input in Java. We will use a very simple way, which is basically the in-built functionality called the Scanner.
What is Scanner?
Let's say, we have various forms or sources, from where we receive data. We can receive data from a Network stream. We can receive stream of data from a file. Or, stream of data coming from console, like Keyboard.
So, there are different kinds of streams from where data can come from. What Scanner does is, it goes and sits over that particular stream from where we want to receive our data. Whatever data is coming from the stream, Scanner scans that data and gives it to us.
So, we will use Scanner to take data input from the user.
Let's see how it's done.
Remember? While declaring a variable, we used to write –
int a = 10;
Similarly, to make a new scanner, we will need to write some more additional things with it,
Scanner sc = new Scanner(System.in);


- Now, here Scanner is acting like a Data type,
- And sc acts like the name of a variable (or name of an Object | which is basically name of our new Scanner).
- To get output displayed on the screen, we use System.out. Similarly, to receive input we use System.in within brackets. Basically we are providing Scanner a stream, from where we are going to receive our data from. It's not going to come from file, nor from the network. It is going to come from the system which is the Keyboard. To, send the output we use System.out, just like that to receive data from console we use System.in .
- This basically is just a way of saying – "We need a new Scanner, which will go and sit on that input stream".
We will get to know about this Scanner in very much detailed manner, when we will be studying Object Oriented Programming.
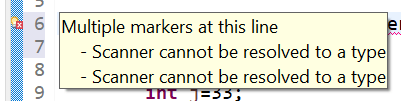
As you can see in Eclipse editor, we are getting an error on line #6 which is 'Scanner cannot be resolved to a type'. It's like our Java compiler is saying – "I don't know what do you mean by this Scanner as I do not have anything called Scanner here."
We did not make Scanner. Scanner is something which is in-built from before.
We just need to direct our compiler where it is built. To do so, we need to include a line at the top at line #1 –
import java.util.Scanner;

NOTE : If your Package's name is default package, you won't have package name displayed at the top of your code in the code editor. In that case, include the line import java.util.Scanner; at the top at line no. 1 of your code. Or you can simply put this line before 'public class ….{'. If you have named your package, let's call it package_name then, at line #1, there will be package package_name; In this case, you need to write this line import java.util.Scanner; at line #2. Or as stated previously, just before this line 'public class ….{'.
As soon as we include this line at the top at line #1, the error goes away. Basically what happened was, on reading this file, compiler understood that to run the code on line #6, one other file is required which is being used here.
We have just imported that file and we can use it now.
Scanner provides us with amazing functionalities where we can tell the Scanner that the data which we are going to receive is an Integer(in our case here).
For that on line #8, #9 and #10, we replace our values with –
sc.nextInt();

- By writing this at line #8, we are telling our Scanner that the data which is going to come will be an integer, bring that integer to us and store that in variable 'i'.
- Similarly, for line #9, another data which is going to come will also be an integer, bring that to us too and store that in variable 'j'.
- Do the same for line #10 too, and store that integer in variable 'k'.
So, while running our program, the program will get stuck at this line #8. This is because, the Scanner waits for the input from the user which it's going to assign to the variable 'i'. So, it's going to stop here and sit, till the user gives some Input.
Let's run our Program.

As we can see, there is nothing on the Console. So, what does that mean? Didn't our program run?
Yes, our program did run. How do we know that?
Can you see that red button like thing on the picture in bottom-right section? This indicates that our program is running, and it is running continuously. The button is basically an option to terminate this program which is running. If we click on that button, our program will terminate.

Let's run our program again.

See, the button turned red again. It simply means our program is running again. But why does it keep running? Simply because it has reached line #8, and it's waiting for an integer input from the user.
From our Keyboard let's give input in console –
- 10
- 20
- 30
And press enter key.

So, we got our output,
- The sum is : 60
- The average is : 20
Variable 'i' became 10, 'j' became 20 and 'k' became 30. These were added and stored in variable 'sum' and accordingly average was stored in variable 'average'.
So, Scanner did the job for us.
Let's try something else. Let's run the program again, the program will get stuck at line #8 waiting for the input.
Here instead of giving the input as some Integer, we provide 'abc' as input, which is not an integer. Let's see what happens.

Scanner will give us an Error – InputMismatchException .
Scanner was hoping that we would give Integer as an Input, but we did not give an Integer. And Scanner is not able to convert 'abc' to an integer. So, that's why Scanner gave us an error here.
Hence the Solution to this problem is :
import java.util.Scanner; public class addNav { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int i=sc.nextInt(); int j=sc.nextInt(); int k=sc.nextInt(); int sum=i+j+k; int average=sum/3; System.out.println("The sum is : " + sum); System.out.println("The average is : " + average); } }
Let us use Scanner in our previous problem of calculating Simple Interest. The modified problem is –
Problem #2
Simple Interest
Print Simple Interest from Principal, Rate of interest and Time.
Here we need to take the input Principal, Rate and Time from the user. Based on these data, we need to calculate the Simple Interest. The formulae for the Simple Interest is –
Simple Interest = (Principal * Rate * Time) / 100
So before, the solution to this problem when we were randomly assigning any values to variables was –
public class simpleInterest { public static void main(String[] args) { int p = 5000; int r = 8; int t = 5; int si = (p * r * t) / 100; System.out.println(si); } }

Let us now –
- import Scanner,
- make a new Scanner, and
- set it up so that it starts taking Input from the user.
Solution :
import java.util.Scanner; public class simpleInterest { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int p = sc.nextInt(); int r = sc.nextInt(); int t = sc.nextInt(); int si = (p * r * t) / 100; System.out.println(si); } }
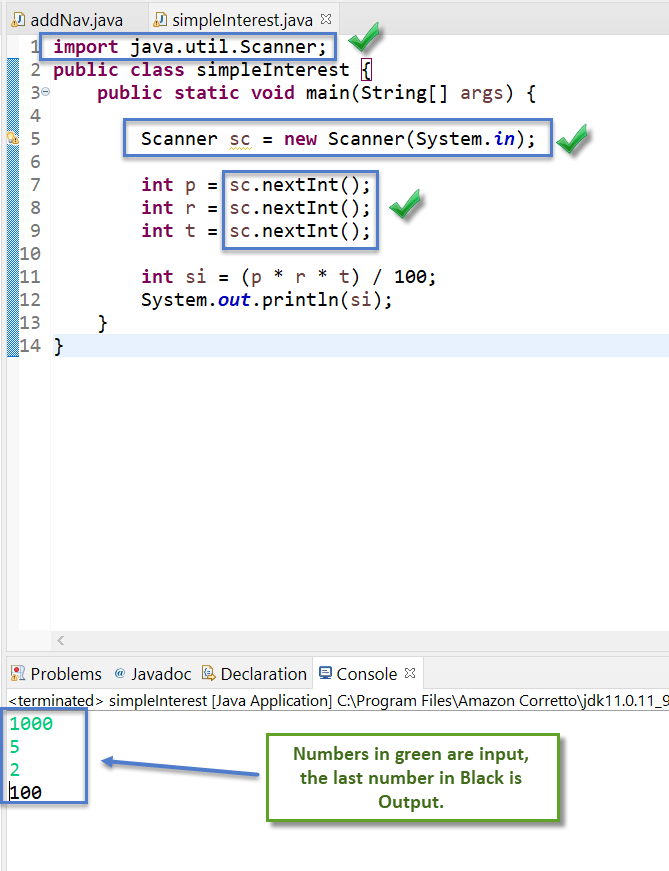
So, as we can see now, our programs have become way more useful compared to what they were before.
That's because our program now does not calculate Simple Interest just based on only fixed values.
Now we can give any values to it, and our program will calculate Simple Interest based on that.
We have seen how to take integer as input. Let's see how to take other data types as input using Scanner. For that I am making a new class called RoughClass.(It's just for understanding purpose, you may not need to create that.)

Take Input of different data types from the user in Java
We will include import java.util.Scanner; at the beginning of our code at line #1.
We will make our new Scanner and name it 'scn' now.

Till now we have seen how to take Integer input of int data type.
int a = scn.nextInt();
For taking an input of data type long , we can write –
long b = scn.nextLong();
For taking an input of data type double , we can write –
double c = scn.nextDouble();
For taking an input of data type float , we can write –
float d = scn.nextFloat();
For taking an input of data type short , we can write –
short e = scn.nextShort();
For taking an input of data type boolean , we can write –
boolean f = scn.nextBoolean();
But, if we want to take an input of data type char , there is nothing called as nextChar();

So, how are we going to take character Input?
A lot of times while coding, we will want to store and use combination of different characters, for example, "pqr".
char data type could only store any one character. Combination of these characters forms what we call as a String .
So, "pqr" is an example of a Reference Data type called String .
Let's say a file has texts in it. And we want to read the text from that file. And that text is basically the combination of characters in it. It's like we will be reading multiple characters at one time. We call these as String.
In Java, just as we can store characters, we can store String too. The syntax to store a String is –
String h = "pqr";
Let's comment out everything in our file and try to print this String.

So, as we can see, we have printed the String which is combination of multiple characters.
Now let's get back to our problem of not being able to take character as input.
We cannot take character as input from the user, but we can take String as an Input from the user.
For taking an input of data type String , we can write –
String h = scn.nextLine();
Let us run this code –

As we can see, our whole line(all the three words) got stored inside our variable 'h' as String.
This is what we achieve using nextLine();
There is one more way by which we can take a String as Input. Instead of nextLine(); we just write next();
String h = scn.next();
Let's try this code –

As we can see in the picture above, only our first word which is 'code' is printed.
nextLine(); accepts the whole line as String. Whereas, next(); breaks the string around the first space, and stores the word before first space as an Input.
Let's learn one more concept.
Since we wrote 'code part time' as input, next(); only used "code" as it's Input in variable 'h'. What about the rest which is " part time"?
Let's include this line and print this after next(); –
String i = scn.nextLine();

So, as seen above, "code" gets stored as String in variable 'h'. And the rest, which is a combination of spaces and characters in it, is stored altogether as String in variable 'i'.
Let's change this line #19, to take integer input from the user instead of String, and run our code –
int i = scn.nextInt();
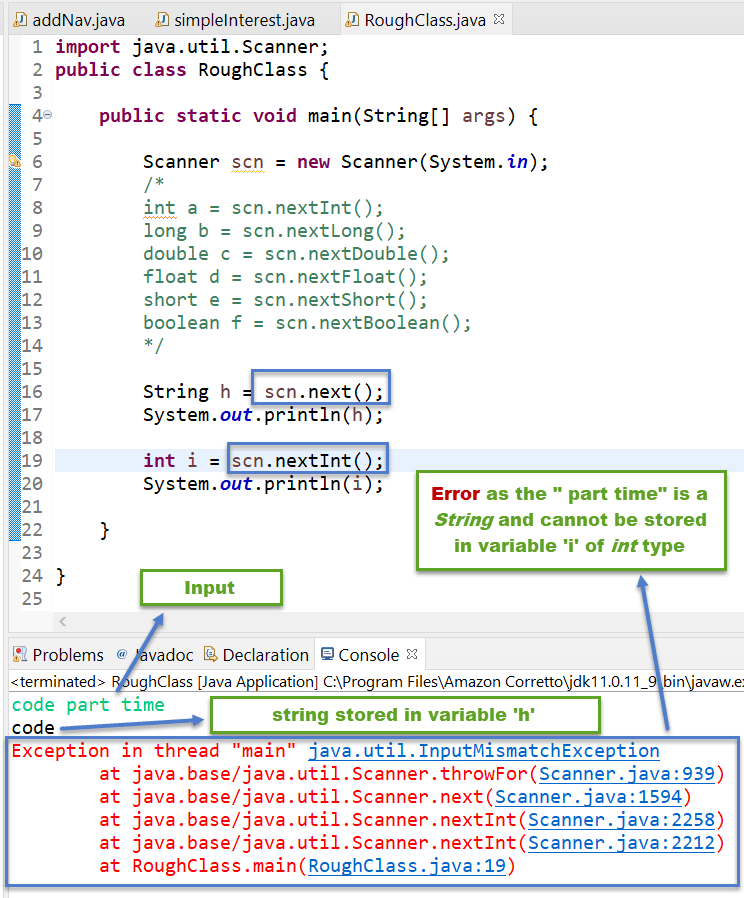
We get the output "code" which is basically the data stored in variable 'h'.
Scanner will try to put the next remaining String " part time" inside variable 'i' which is of type int, and since storing of a string in int is not possible, we will get an InputMismatchException Error.
So, we can take String input which is multiple characters combined.
But what if we want only a character as an Input instead of String?
For this, we will first take input as String, and from this String, we will tell the system which position's character we want.
Let's say, we have a string called "code". If we want the first character from this String, we will write the code –
char i = h.charAt(0);
If we want the second character in another variable, we will write the code –
char j = h.charAt(1);
If we want the third character in another variable, we will write the code –
char k = h.charAt(2);
Just remember, the initial numbering starts with 0.
First character is called 0th Character, Second character is called 1st Character and so on.
And this is how we get the Output.

So, if we want a character as an Input, we will take it as a String from the user, and we will then extract the 0th character out of it using charAt(posn).
Codes in RoughClass.java –
import java.util.Scanner; public class RoughClass { public static void main(String[] args) { Scanner scn = new Scanner(System.in); /* int a = scn.nextInt(); long b = scn.nextLong(); double c = scn.nextDouble(); float d = scn.nextFloat(); short e = scn.nextShort(); boolean f = scn.nextBoolean(); */ String h = scn.next(); System.out.println(h); char i = h.charAt(0); System.out.println(i); char j = h.charAt(1); System.out.println(j); char k = h.charAt(2); System.out.println(k); } }
This is how we take Input from the user in Java.
Types of Input in Java
Input refers to any information or data sent to a computer for processing.
Java allows us to insert data or input in a variety of ways. In Java, data can be entered or inserted using the following methods:
- Initialization of Variables
- Parameterised Input
- Scanner Input
Initialization of Variables
The assignment of an initial value to a variable at declaration is known as initialization.
- Variables are initialised during declaration in this method. For example,
int a=5;
In this statement, 5 is initially placed in a memory location defined by the variable a.
- It's also possible that initialization is a reference to another variable. For example,
int b=a;
The value of a is dynamically initialised in b. It's worth noting that whatever the value of a is, it's the initial value of b.
- A call to a method can also be used to signify initialization. For example,
double s= Math.sqrt(9);
The integer 3.0, which is the square root of 9, is used as the initial value for s. As can be seen, a method (Math.sqrt()) can be called during initialization as well.
Parameterised Input
Parameterised input refers to the process of passing values to arguments in a method as it is being executed.
Scanner Input
The Scanner class is a java.util package class that allows you to read values of various types from the keyboard or a file.
As the name implies, a package is a collection of pre-compiled classes, interfaces, and other components.
System.in is an InputStream object that is often attached to a console program's keyboard input.
The following table lists some of the scanner class's most common functions and methods.
Member Methods of the Scanner Class | Usage |
---|---|
nextShort() | It accepts an integer from the keyboard of short data type. |
nextInt() | It accepts an integer from the keyboard of int data type. |
nextLong() | It accepts an integer from the keyboard of long data type. |
nextFloat() | It accepts a decimal number from the keyboard of float data type. |
nextDouble() | It accepts a decimal number from the keyboard of double data type. |
next() | It accepts a single word from the keyboard of String data type. |
nextLine() | It accepts a single line of text (i.e, a sentence) from the keyword of String data type. |
next().charAt(0) | It accepts a single character from the String of char data type. |
Errors in Java
It's always possible that an error will appear in a program when we're typing it, or when we're thinking about how to solve a problem.
This could result in an unexpected result or no outcome at all.
As a result, it's critical to recognize and manage all possible error conditions in a program so that it doesn't crash or terminate during operation.
A bug is a flaw, failure, or error in a program or system that causes it to generate an inaccurate or unexpected result, or to act in unexpected ways.
A bug in a program is also known as an error, and the process of fixing it is known as debugging.
Types of Errors in Java
There are three types of errors that can generally occur in a Java program :
- Syntax errors
- Logical errors
- Run-time errors
Syntax Errors
A syntax error occurs when the syntax of a sequence of characters or tokens intended to be written in a certain program is incorrect.
When a syntax error occurs, the compiler will stop translating the source code to byte code until the error is corrected.
Example:
float k = 12.3;
These type of errors generally occur because of –
- Missing semicolon.
- Mismatched braces in classes and methods.
- Wrongly accessed variable, whose visibility doesn't exist.
- Misspelled keywords and identifiers.
- Missing quotes in a character/string.
- Incompatible types in assignments/initialization.
- Bad reference to objects.
- Package name improperly given in the import statement.
Logical Errors
A logical error is a bug in a program that causes it to behave strangely but not to terminate completely (or crash).
A user-written program may be syntactically correct, yet it does not execute as intended, i.e., the logic of the program may be flawed.
In such circumstances, the program produces entirely incorrect results. As a result, even though a program is syntactically correct, it may not work for its intended purpose.
This is due to a logical or methodological error in the solution.
Example:
int i=24; int j=33; int sum=i-j; System.out.println("The sum of two numbers is : " + sum);
Run Time Errors
A run time error is an error that occurs during the execution of a program.
A program may compile correctly and appear to have perfect logic at times. But certain aberrant conditions in the code sequence may arise during run time, i.e. during execution, resulting in a run-time error.
However, such errors do not invariably lead to more errors.
Example :
int i=sc.nextInt(); int j=sc.nextInt(); int rem = i/j; System.out.println("The div is : " + rem);
The commonly run-time errors are often because of the following reasons:
- Dividing an integer by zero.
- Accessing an element that is out of bounds of an array. {We will learn about Arrays as we proceed further.}
- Trying to store a value which is incompatible a certain data-type.
- Attempting to use a negative index for an array.
- Converting an invalid string to a number.
- Accessing a character that is out-of-bounds of a string.
You may like to Explore –
How to obtain substring of a string in Java?
Understanding Modulus Operator (Modulo or Remainder) in Java
Cheers.
Happy Coding.

Authored by Rawnak
Founder and lead author of CodePartTime.com.
Source: https://codeparttime.com/take-input-from-the-user-in-java-errors-in-java/